※ GoogleMapAPI のキー発行、VueCLI のインストールなどはあらかじめ終わっているものとします
vue プロジェクトを作成します。
vue create google_map_sample
preset は Default ([Vue 2] babel, eslint)
を選択します。
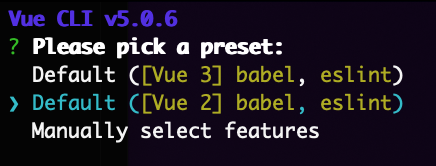
npm で vue-google-maps をインストールします。
npm install vue2-google-maps
main.js を書き換えます。
import Vue from 'vue'
import App from './App.vue'
import * as VueGoogleMaps from 'vue2-google-maps'
Vue.config.productionTip = false
Vue.use(VueGoogleMaps, {
load: {
key: 'キー',
libraries: 'places',
region: 'JP',
language: 'ja'
}
});
new Vue({
render: h => h(App)
}).$mount('#app')
App.vue を書き換えます。
<template>
<div id="app">
<HelloWorld msg="Welcome to Your Vue.js App"/>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
components: {
HelloWorld
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
HelloWorld.vue を書き換えます。
<template>
<div>
<GmapMap
:center='center'
:zoom='15'
ref="myMapRef"
:options="{
streetViewControl: false,
mapTypeControl: false,
fullscreenControl: false
}"
style="width: 100%; height: 500px"
@dragend="onDragEnd"
>
<GmapMarker v-for="m in markers"
:position="m.position"
:title="m.title"
:clickable="true"
:draggable="false"
:key="m.id">
</GmapMarker>
</GmapMap>
</div>
</template>
<script>
import {gmapApi} from 'vue2-google-maps'
export default {
name: 'GoogleMap',
data() {
return {
center: {
lat: 35.6809591,
lng: 139.7673068
},
geocoder: null,
markers: []
}
},
computed: {
google: gmapApi
},
mounted() {
this.$gmapApiPromiseLazy().then(() => {
this.geocoder = new this.google.maps.Geocoder()
this.mapSearch('コンビニ');
})
},
methods: {
onDragEnd: function() {
const map = this.$refs.myMapRef.$mapObject;
this.center.lat = map.center.lat();
this.center.lng = map.center.lng();
},
mapSearch: function(keyword) {
const request = {
location: this.center,
radius: 1000,
keyword: keyword
};
const map = this.$refs.myMapRef.$mapObject;
const service = new this.google.maps.places.PlacesService(map);
this.markers = [];
service.nearbySearch(request, function(results, status) {
if (status === this.google.maps.places.PlacesServiceStatus.OK) {
results.forEach(place => {
let marker = {
position: place.geometry.location,
icon: place.icon,
title: place.name,
id: place.place_id
};
this.markers.push(marker);
});
}
}.bind(this));
}
}
};
</script>
npm でローカルサーバを立ち上げます。
npm run serve
http://localhost:8080/ にアクセスすると、東京駅周辺のコンビニがピンで表示されました。
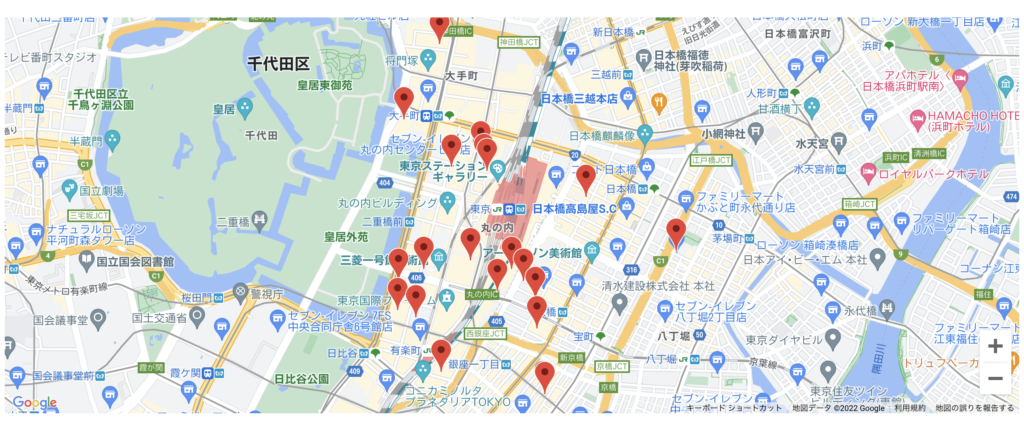
ちなみに検索結果は 20 件のようです。
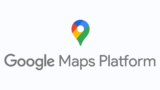
プレイス ライブラリ | Maps JavaScript API | Google for Developers
デフォルトでは、各 Place Search は 1 つのクエリに対して最大 20 件の結果を返します。ただし、各検索では、3 ページに分けて最大 60 件の結果を返すことができます。
https://developers.google.com/maps/documentation/javascript/places?hl=ja#PlaceSearchPaging